Dart has emerged as a powerful language for backend development, thanks to frameworks like shelf
and dartstream
. However, deploying a Dart backend efficiently requires a reliable and scalable approach. This is where Docker comes in.
Docker allows you to package your Dart application with all its dependencies into a lightweight, portable container. This ensures consistency across different environments, making deployment seamless and hassle-free.
In this tutorial, we'll explore the step-by-step process of containerizing a Dart backend application using Docker—from setting up the environment to optimizing and deploying it on the cloud. Whether you're new to Docker or looking to streamline your deployment workflow, this guide will help you get your Dart backend up and running smoothly.
Table of Contents
- Prerequisites
- Preparing the Dart Backend Application
- Writing a Dockerfile for the Dart Backend
- Building and Running the Docker Container Locally
- Deploying the Dockerized Dart Backend
- Conclusion
Prerequisites
Before diving into deploying Dart backend apps with Docker, you'll need to set up your development environment with several key tools and understand some basic concepts. This foundation will ensure a smooth workflow throughout the containerization and deployment process.
Dart SDK Installation
First, you'll need the Dart SDK installed on your development machine. Visit the official Dart website (dart.dev) and follow the installation instructions for your operating system. Verify your installation by running dart --version
in your terminal.
For this tutorial, we recommend using Dart 3.0 or newer to take advantage of the latest language features.
Docker Installation
Docker is essential for our containerization workflow. Download and install Docker Desktop (for Windows and macOS) or Docker Engine (for Linux) from docker.com. After installation, verify that Docker is running properly with the command docker --version
. Ensure you have permissions to run Docker commands without sudo (on Linux systems) to avoid permission issues during development.
To check if Docker is running correctly, execute the command docker run hello-world
. If the test container runs successfully, your Docker installation is ready.
With your environment all set up, you're ready to containerize your Dart backend application!
Preparing the Dart Backend Application (Using shelf
)
Before we containerize our Dart backend, we need to set up a simple server using the shelf
package. shelf
is a lightweight web server framework that makes it easy to build and serve HTTP APIs in Dart.
Creating a Dart Project
- Initialize a New Dart Project
Open a terminal and run:
dart create dart_backend
This creates a new Dart project named dart_backend
.
- Navigate to the Project Directory
cd dart_backend
Adding Shelf Dependencies
To use shelf
, add it to the project’s dependencies:
dart pub add shelf
dart pub add shelf_router
shelf
provides the core HTTP server functionality.shelf_router
makes it easier to define API routes.
Writing a Simple Shelf Server
Now, let's create a basic server file. Open bin/server.dart
and replace its contents with the following code:
import 'dart:io';
import 'package:shelf/shelf.dart';
import 'package:shelf/shelf_io.dart' as shelf_io;
import 'package:shelf_router/shelf_router.dart';
// Define a simple router with basic routes
final router = Router()
..get('/', (Request request) => Response.ok('Hello, Dart Backend!'))
..get('/hello/<name>', (Request request, String name) =>
Response.ok('Hello, $name!'));
// Middleware to log requests
Middleware logRequests = (Handler innerHandler) {
return (Request request) async {
print('Request: ${request.method} ${request.url}');
return innerHandler(request);
};
};
void main() async {
final handler = Pipeline().addMiddleware(logRequests).addHandler(router);
final server = await shelf_io.serve(handler, InternetAddress.anyIPv4, 8080);
print('Server running on http://${server.address.host}:${server.port}');
}
Running the Dart Backend Locally
Start the server by running:
dart run bin/server.dart
If everything is set up correctly, you should see output like:
Server running on http://0.0.0.0:8080
Test it by visiting:
- http://localhost:8080/ → Returns "Hello, Dart Backend!"
Handling Configuration and Environment Variables
Instead of hardcoding values, use environment variables for better flexibility.
- Add the
dotenv
package
dart pub add dotenv
- Create a
.env
file in the project root:
PORT=8080
- Load Environment Variables in
server.dart
import 'package:dotenv/dotenv.dart';
void main() async {
// Load environment variables from .env file
var env = DotEnv(includePlatformEnvironment: true)..load();
// Get PORT from environment variables, with fallback to 8080
final port = int.parse(env['PORT'] ?? '8080');
final handler = Pipeline().addMiddleware(logRequests).addHandler(router);
final server = await shelf_io.serve(handler, InternetAddress.anyIPv4, port);
print('Server running on http://${server.address.host}:${server.port}');
}
With our Dart backend is ready, the next step is containerizing it with Docker.
Writing a Dockerfile for the Dart Backend
Our Dart backend is ready, we need to containerize it using Docker. A Dockerfile defines how our Dart application will be built and run inside a container. Below, we’ll create a simple yet efficient Dockerfile
for our Dart backend.
Step 1: Choosing a Base Image
We need a Dart-compatible base image. The official Dart SDK image is a good choice:
dart:stable
→ Includes the full Dart SDKdart:stable-runtime
→ Includes only the Dart runtime (smaller, for production use)
For a smaller and optimized final image, we’ll use multi-stage builds—starting with dart:stable
for building and then dart:stable-runtime
for running.
Step 2: Creating the Dockerfile
Inside the project root, create a Dockerfile
and add the following contents:
# === Stage 1: Build ===
FROM dart:stable AS build
# Set working directory inside the container
WORKDIR /app
# Copy pubspec files and resolve dependencies
COPY pubspec.* ./
RUN dart pub get
# Verify dependencies are installed
RUN ls /root/.pub-cache/hosted/pub.dev
# Copy the rest of the application
COPY . .
# Ensure dependencies are available after copying source code
RUN dart pub get
# Compile the Dart script to native executable
RUN dart compile exe bin/server.dart -o server
# === Stage 2: Runtime ===
FROM debian:bullseye-slim AS runtime
# Set working directory
WORKDIR /app
# Install necessary runtime dependencies
RUN apt-get update && apt-get install -y ca-certificates && rm -rf /var/lib/apt/lists/*
# Copy only the compiled binary from the build stage
COPY --from=build /app/server .
# Expose the application port
EXPOSE 8080
# Command to run the server
CMD ["./server"]
Step 3: Explaining the Dockerfile
Stage 1 (Build Stage)
- Uses the full Dart SDK (
dart:stable
) - Installs dependencies
- Copies the application files
- Compiles the Dart backend into a native executable for better performance
Stage 2 (Runtime Stage)
- Uses
debian:bullseye-slim
for the runtime—a lightweight alternative to keep the image small. - Copies only the compiled binary, reducing the final image size
- Exposes port
8080
- Runs the compiled
server
binary
Step 4: Expose Ports and Set the Entry Point
EXPOSE 8080
→ Opens port 8080 for incoming requestsCMD ["./server"]
→ Defines the command to run the server
Now that the Dockerfile
is ready, we'll build and run the container locally in the next section.
Building and Running the Docker Container Locally
Once the Dockerfile
is ready, the next step is to build the Docker image and run the container locally to ensure everything works correctly. This process involves compiling the Dart backend into a self-contained Docker image and launching it as a container.
Step 1: Build the Docker Image
Run the following command in the terminal from the root of your project directory:
docker build -t dart-backend .
Here’s what happens during this step:
- Docker reads the
Dockerfile
and follows the instructions to set up the environment. - The Dart application is compiled into a native executable.
- The final runtime image is created using a minimal base image.
You should see a similar output on your terminal:
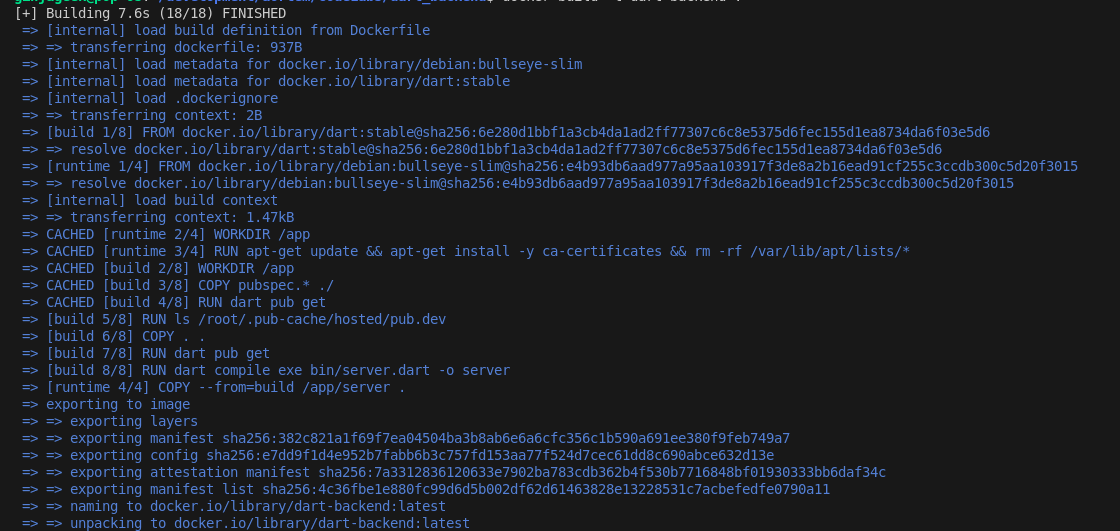
Step 2: Run the Docker Container
Once the image is built, start a container using:
docker run -p 8080:8080 dart-backend
Explanation of the flags:
-p 8080:8080
maps port 8080 inside the container to port 8080 on the host machine.dart-backend
is the name of the Docker image.
Step 3: Verify the Running Container
After running the container, check if it’s working by opening a browser or using curl
:
curl http://localhost:8080
If everything is set up correctly, you should see a response like:
Hello, Dart Backend!
Step 4: List Running Containers
docker ps
The output on your terminal should look like this:

Step 5: Stop the Running Container
To stop the container, first find its CONTAINER ID
using docker ps
, then run:
docker stop <CONTAINER_ID>
Deploying the Dockerized Dart Backend
Once the Dart backend is successfully containerized and tested locally, the next step is deploying it to a cloud platform. This section outlines how to push the Docker image to a registry and deploy it to a cloud service.
Pushing the Docker Image to a Registry
Before deployment, the Docker image needs to be uploaded to a container registry such as Docker Hub, Google Container Registry (GCR), or Amazon Elastic Container Registry (ECR).
Step 1: Login to Docker Hub (or another registry)
docker login -u <your-username>
If that was successful, you'll get a feedback:
Login Succeeded
Step 2: Tag the Docker Image
Tagging a docker image follows the syntax:
docker tag SOURCE_IMAGE[:TAG] TARGET_IMAGE[:TAG]
If deploying to Docker Hub, tag the image with your repository name for the new image as follows:
docker tag dart-backend <your-dockerhub-username>/dart-backend:latest
Step 3: Push the Image to the Registry
docker push <your-dockerhub-username>/dart-backend:latest
If using another registry, update the tag accordingly before pushing.
Deploying to a Cloud Platform
Several cloud services support Dockerized applications, including AWS (ECS, Fargate, EC2), Google Cloud Run, etc.
Option 1: Deploy to Google Cloud Run
Google Cloud Run is a fully managed service that allows running containers with minimal configuration.
- Enable Cloud Run on Google Cloud
gcloud services enable run.googleapis.com
- Deploy the Docker Image to Cloud Run
gcloud run deploy dart-backend \
--image gcr.io/<your-project-id>/dart-backend:latest \
--platform managed \
--region us-central1 \
--allow-unauthenticated
- Once deployed, Google Cloud Run provides a public URL to access your API.
Option 2: Deploy to AWS ECS (Fargate)
Amazon ECS with Fargate is a great serverless option for running Docker containers.
- Create a Repository in AWS ECR
aws ecr create-repository --repository-name dart-backend
- Tag the Image for AWS ECR
docker tag dart-backend <aws-account-id>.dkr.ecr.<region>.amazonaws.com/dart-backend:latest
- Push the Image to ECR
docker push <aws-account-id>.dkr.ecr.<region>.amazonaws.com/dart-backend:latest
Verify the Deployment
Once the container is running in the cloud, test the deployment by accessing the provided public URL:
curl https://your-cloud-url.com
You should get the response:
Hello, Dart Backend!
Conclusion
Dockerizing and deploying a Dart backend provides a robust, scalable, and efficient way to run your application across different environments. In this guide, we walked through the step-by-step workflow—from setting up the development environment, preparing the Dart backend with Shelf, writing a Dockerfile, handling environment variables, and building and running the container locally, to finally deploying it to a cloud service.
By containerizing the Dart backend, we ensure consistency across development, testing, and production environments, making deployments more predictable and manageable. Whether you're hosting on Docker Hub, AWS, or another cloud provider, this workflow sets a solid foundation for running your Dart applications in a modern, cloud-native way.
Now that you have a fully Dockerized Dart backend, you can explore scaling with Kubernetes, automating deployments with CI/CD, or integrating with other microservices.